Client SDK
ePac Node.js SDK
The ePac Node.js SDK is a powerful and straightforward tool for building ePac applications.
Up and running
As previously mentioned, create-ePac-app can generate an express-based app, which serves as an excellent starting point for building your application.
However, if you prefer to use a different HTTP server or need to integrate ePac webhooks into an existing Node.js application, you can simply install @ePac/node-client in your application.
npm install --save @ePac/node-client
If your application only needs to respond to webhooks, you can include it in the following manner.
const {WebhookResponse} = require('@ePac/node-client');
If your application requires making REST API calls, you can include it in the following manner.
const ePac = require('@ePac/node-client');
const {WebhookResponse} = ePac;
const client = ePac('your-account-sid', 'your-api-key', {baseUrl: 'https://ePac.epacific.net/api/'});
Please note that, you should replace https://ePac.epacific.net in the above example with the appropriate URL of your own ePac system.
How to use it - Webhooks
Responding to webhooks using the ePac Node.js SDK is a straightforward process. Once you have included the SDK in your application, you can respond to webhooks by defining a function that takes a webhook request and returns a response. The SDK provides several utility functions to help you parse and manipulate the request and response objects.
router.post('/', (req, res) => {
const app = new WebhookResponse();
app.pause({length: 1.5});
app.say({text 'hi there, and welcome to ePacific!'});
res.status(200).json(app);
});
The WebhookResponse object provides methods for each of the ePac verbs. For instance, you can check out the available options for the pause verb here, and for the say verb here. Once you have added all the verbs that you want to execute, in the order you want them to happen, simply return the app object in the 200 OK response to the HTTP request.
How to use it - REST API
To make a REST API call, you can use the client object that was created earlier, as shown below.
await client.calls.update(req.body.call_sid, {mute_status: 'mute'});
You can find the documentation for the REST API here.
Create a new ePac app
To create a new ePac webhook or REST API application, the easiest method is to use the create-ePac-app command. However, it is necessary to ensure that your system has Node version 10.16 or higher and npm version 5.6 or higher. To initiate a project, execute the following command:
npx create-ePac-app my-app
cd my-app
npm start
To gain a better understanding of the functionality offered by create-ePac-app, you can execute it with the –help flag. This will display the available options and help you explore its capabilities in more detail.
npx create-ePac-app --help
----
Usage: create-ePac-app [options] project-name
Options:
-v, --version display the current version
-s, --scenario <scenario> generates sample webhooks for specified scenarios, default is dial and tts
-h, --help display help for command
Scenarios available:
- tts: answer call and play greeting using tts,
- dial: use the dial verb to outdial through your carrier,
- record: record the audio stream generated by the listen verb,
- auth: authenticate sip devices, or
- all: generate all of the above scenarios
Example:
$ npx create-epac-app -s "all" my-app
create-ePac-app is a tool that scaffolds an ePac application for you, utilizing express as the http middleware. It comes with default configuration, generating three endpoints:
/ hello-world: A webhook that utilizes text-to-speech to greet the user.
/ dial-time: A webhook that greets the user and then dials to a UK-based speaking clock.
/ call-status: A webhook that records call status events.
These basic webhook apps are especially beneficial for testing new systems or accounts, and for verifying that carrier interconnects and text-to-speech are functioning correctly.
Certain webhooks created via this tool require environment variables to be set, and a pm2 ecosystem file with placeholders is generated by default, which you can fill out as per your requirement.
Additional scenarios
Depending on the options you provide, create-ePac-app can generate additional webhooks beyond the default ones.
Record
One of the additional webhooks that create-ePac-app can generate is a webhook application that employs the “listen” verb to transmit a real-time audio stream to an endpoint that the application also exposes. To achieve this, you will need to supply the path to the websocket server as an environment variable, along with an AWS S3 bucket that the application can upload recordings to. It is important to note that the specified bucket must be both public and writable by all.
Once the application is configured, it will prompt the caller to record a message, and the recorded message will be stored in S3 storage. It is worth noting that this is just one example of how to store recordings, and you can use any storage backend that meets your application’s requirements.
Authenticate SIP users
create-ePac-app can also generate a webhook application that demonstrates how to authenticate sip users and webrtc clients. The application uses an in-memory database of sip usernames and passwords, which is a straightforward example. However, it is important to note that you can implement your own database or other storage backend to hold authentication credentials based on your specific requirements.
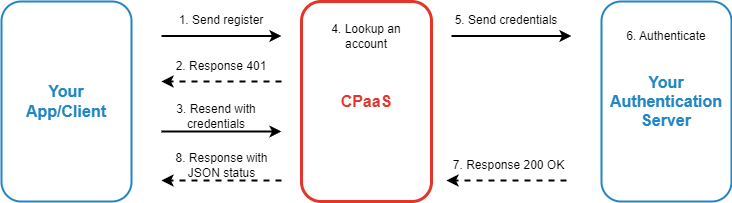
SIP clients such as softphones, SIP phones, and webrtc clients register with the ePac platform to make and receive calls. ePac uses webhooks to notify the application of incoming REGISTER or INVITE requests.
When ePac receives an incoming SIP request from an endpoint that is not a carrier SIP trunk, the request is challenged with a 401 Unauthorized response that includes a WWW-Authenticate header.
The originating SIP device then resends the request with credentials (e.g. an Authorization header).
ePac retrieves the SIP domain from the request and uses it to lookup the account that owns that domain.
The associated registration webhook is invoked with the details provided in the Authorization header.
The application retrieves the password associated with the username and performs digest authentication to authenticate the request using the information provided.
The application responds with a 200 OK to the HTTP POST, regardless of whether the request is authenticated or not.
The SIP device will receive the status, if:
The request is authenticated, the application responds with a JSON body containing a status attribute with a value of “ok”. Optionally, the application may include an expiration value in the response to enforce a shorter expiration time.
The request is not authenticated, the application responds with a JSON body containing a status of “fail” and an optional msg attribute indicating the reason for authentication failure.
Exposing your endpoints for testing
If you do not have a server with a public address to host your ePac application, you can use ngrok as a workaround. The application will be listening on port 3000 by default (which can be changed in the ecosystem file), so after installing ngrok, you can generate a public URL for the application. This enables you to test your application and receive external requests even if your server does not have a public IP address.
Please contact us for timely support via:
Phone: 1900-1563
Email: support@epacific.com.vn